API Scenario
Auto Hanging
import utility_api
import ApiTypes
print("API Test Scenario - Auto Hanging")
#Adding Path for Garment and Hanger
garmentPath = utility_api.GetCLOAssetFolderPath(True) + "Garment/Male_T-shirt.zpac" # change this to a path to your garment
hangerPath = utility_api.GetCLOAssetFolderPath(True) + "Avatar/Hanger/Adult_Shirt_Hanger_V3.avt" # change this to a path to your hanger.
#Option for importing OBJ
objoption = ApiTypes.ImportExportOption()
objoption.scale = 1 # specify this if your hanger is in OBJ. For example, 1 means 1mm scale. 10 means 10cm scale.
#Auto Hanging API
utility_api.AutoHang(garmentPath, hangerPath, 0, objoption) # toggle the third input - 0 for top hangers, 1 for bottom hangers
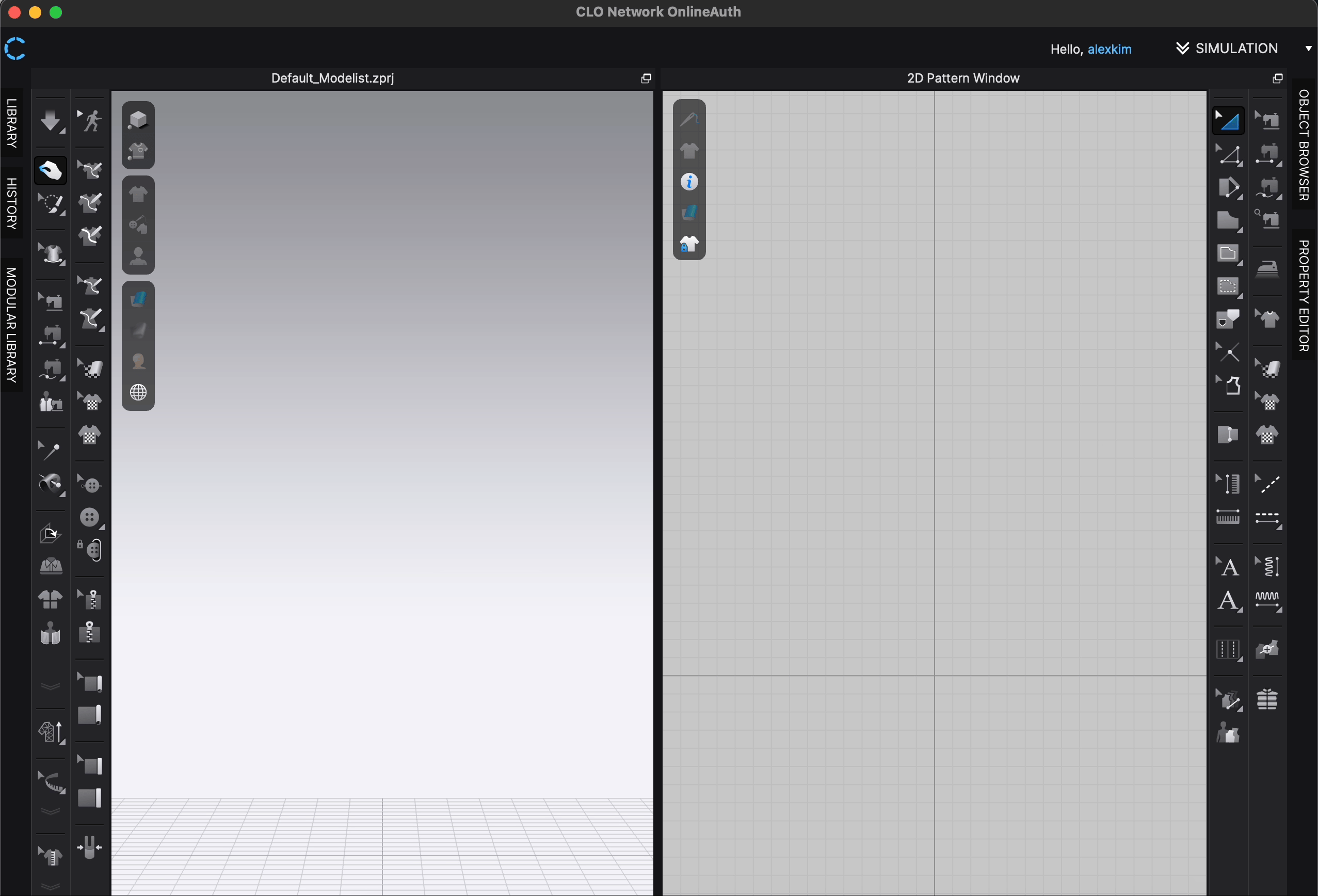
Please Download the Auto Hanging Sample Sample Python Script Here -> S3 , OSS
Simulation
import import_api
import utility_api
print("API Test Scenario - Simulation")
cloAssetFolderPath = "C:/Users/Public/Documents/CLO/Assets/Avatar/Avatar/"
cloGarmentFolderPath = "C:/Users/Public/Documents/CLO/Assets/Garment/"
# Import Avatar File(avt)
avtfilePath = cloAssetFolderPath + "/Female_V2/FV2_Feifei.avt"
import_api.ImportFile(avtfilePath)
# Import Cloth File(zpac)
zpacfilePath = cloGarmentFolderPath + "/Female_T-shirt.zpac"
import_api.ImportFile(zpacfilePath)
# Simulation
utility_api.Simulate(100)
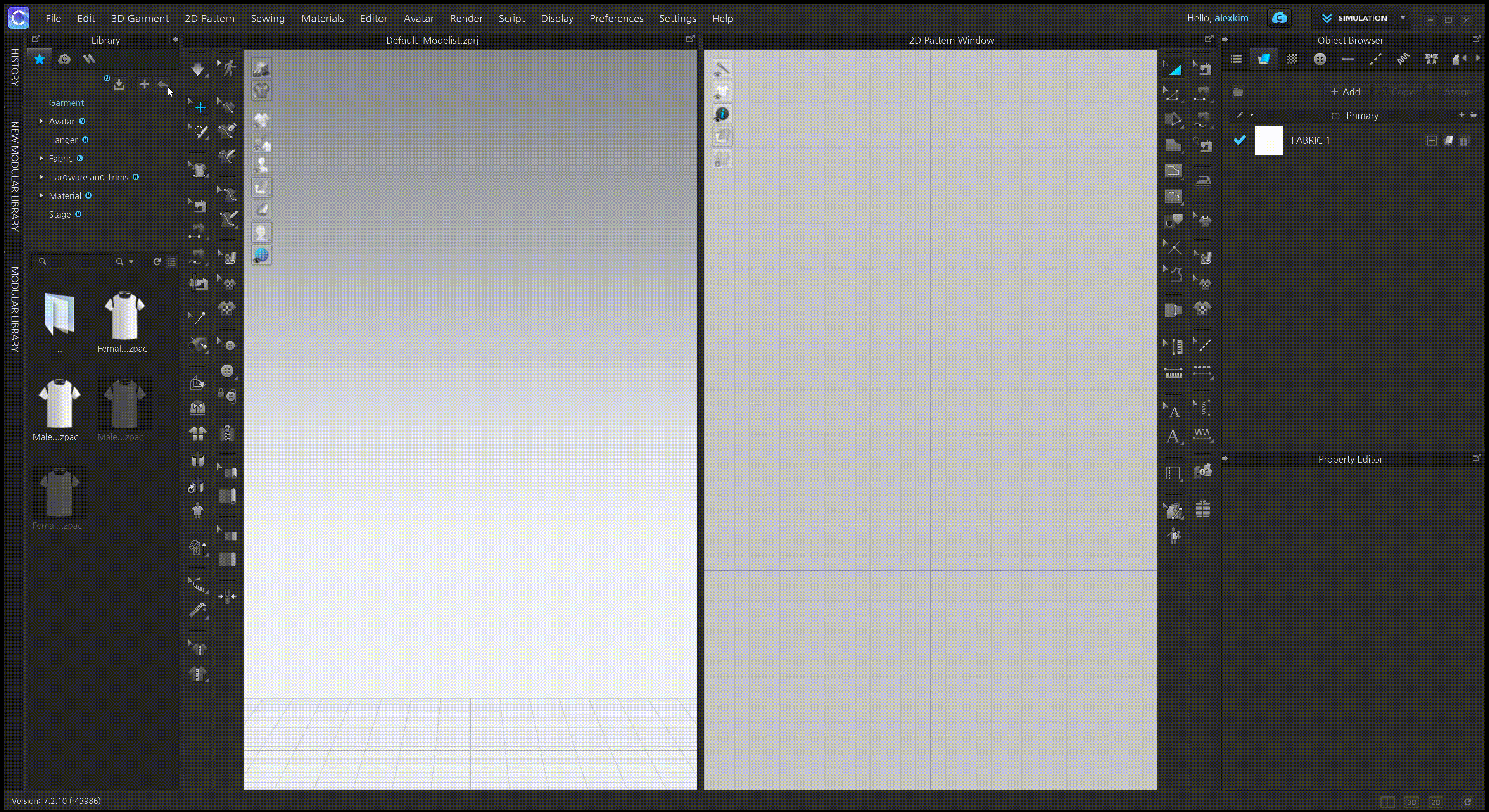
Rendering
import import_api
import utility_api
import fabric_api
import pattern_api
import export_api
print("API Test Scenario - Rendering")
cloAssetFolderPath = "C:/Users/Public/Documents/CLO/Assets"
# Import Avatar File(avt)
avtfilePath = cloAssetFolderPath + "/Avatar/Avatar/Female_V2/FV2_Feifei.avt"
import_api.ImportFile(avtfilePath)
# Import Cloth File(zpac)
zpacfilePath = cloAssetFolderPath + "/Garment/Female_T-shirt.zpac"
import_api.ImportFile(zpacfilePath)
# Simulation
utility_api.Simulate(100)
# Import Fabric(zfab)
zfabFilePath = cloAssetFolderPath + "/Materials/Fabric/Cotton_Oxford.zfab"
addedFabricIndex = fabric_api.AddFabric(zfabFilePath)
# Assign Fabric to Pattern
patternCount = pattern_api.GetPatternCount()
for i in range(patternCount):
fabric_api.AssignFabricToPattern(addedFabricIndex, i)
# Rendering
savedFilepathList = export_api.ExportRenderingImage(False)
print(savedFilepathList) #utility_api.GetCLOTemporaryFolderPath()
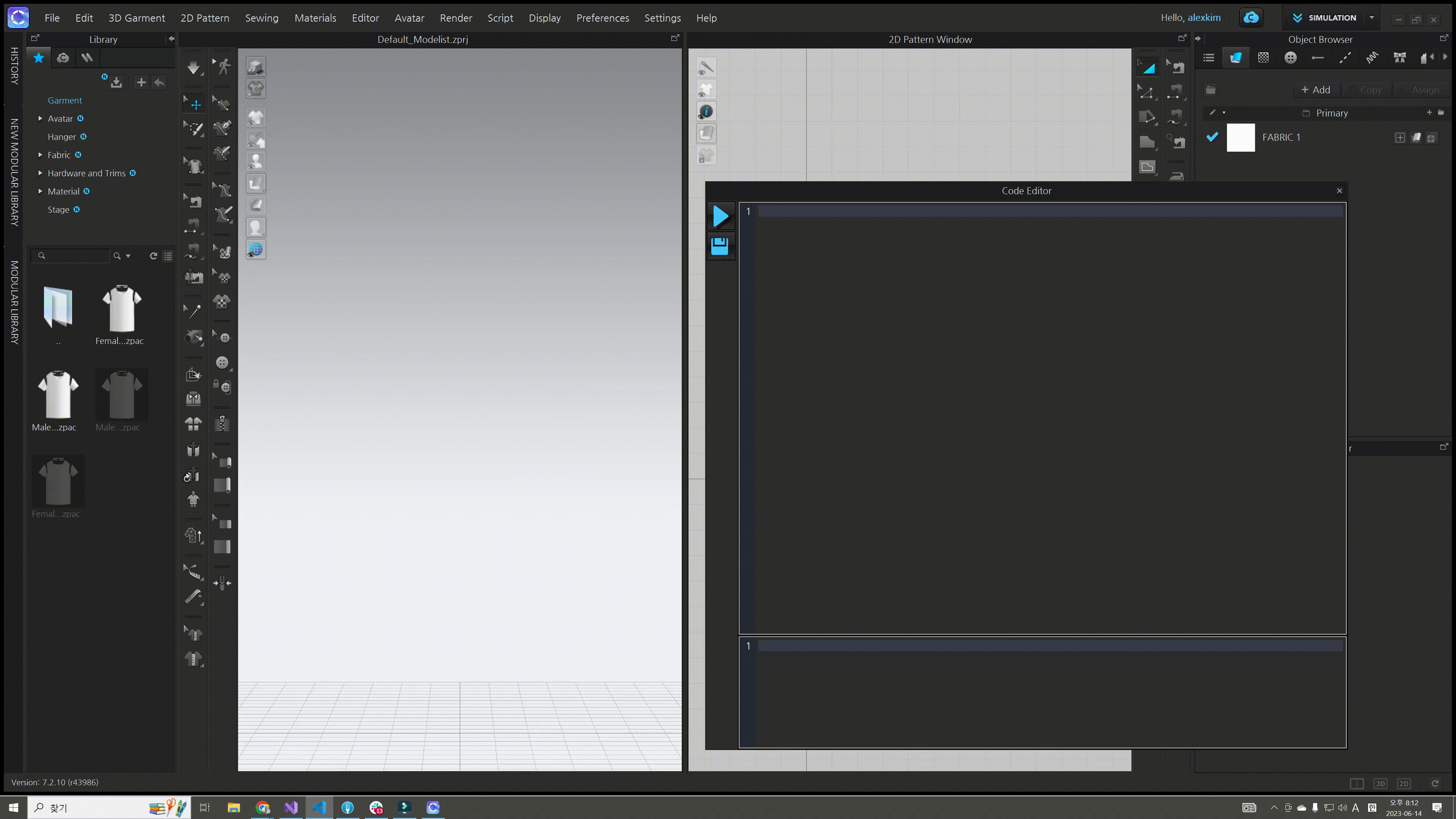
Pattern
import import_api
import utility_api
import fabric_api
import pattern_api
import export_api
print("API Test Scenario - Pattern")
# init rectangle
rectPoints = (
(0.0, -300.0, 0)
, (0.0, -200.0, 0)
, (100.0, -200.0, 0)
, (100.0, -300.0, 0)
)
pattern_api.CreatePatternWithPoints(rectPoints)
# free polygon
freePolygonPoints = (
(0.0, 0.0, 0)
, (0.0, 100.0, 3)
, (100.0, 100.0, 0)
, (200.0, 100.0, 3)
, (150.0, 50.0, 3)
, (200.0, 50.0, 0)
, (300.0, 50.0, 3)
, (250.0, 100.0, 3)
, (300.0, 100.0, 0)
, (350.0, 100.0, 3)
, (300.0, 50.0, 0)
, (150.0, 0.0, 2)
)
pattern_api.CreatePatternWithPoints(freePolygonPoints)
# half circle
halfCirclePoints = (
(0.0, 200.0, 0)
, (0.0, 300.0, 3)
, (100.0, 300.0, 0)
, (200.0, 300.0, 3)
, (200.0, 200.0, 0)
)
pattern_api.CreatePatternWithPoints(halfCirclePoints)
#Path
cloAssetFolderPath = "C:/Users/Public/Documents/CLO/Assets"
# Import Fabric(zfab)
zfabFilePath = cloAssetFolderPath + "/Materials/Fabric/Cotton_Oxford.zfab"
addedFabricIndex = fabric_api.AddFabric(zfabFilePath)
# Assign Fabric to Pattern
patternCount = pattern_api.GetPatternCount()
for i in range(patternCount):
fabric_api.AssignFabricToPattern(addedFabricIndex, i)
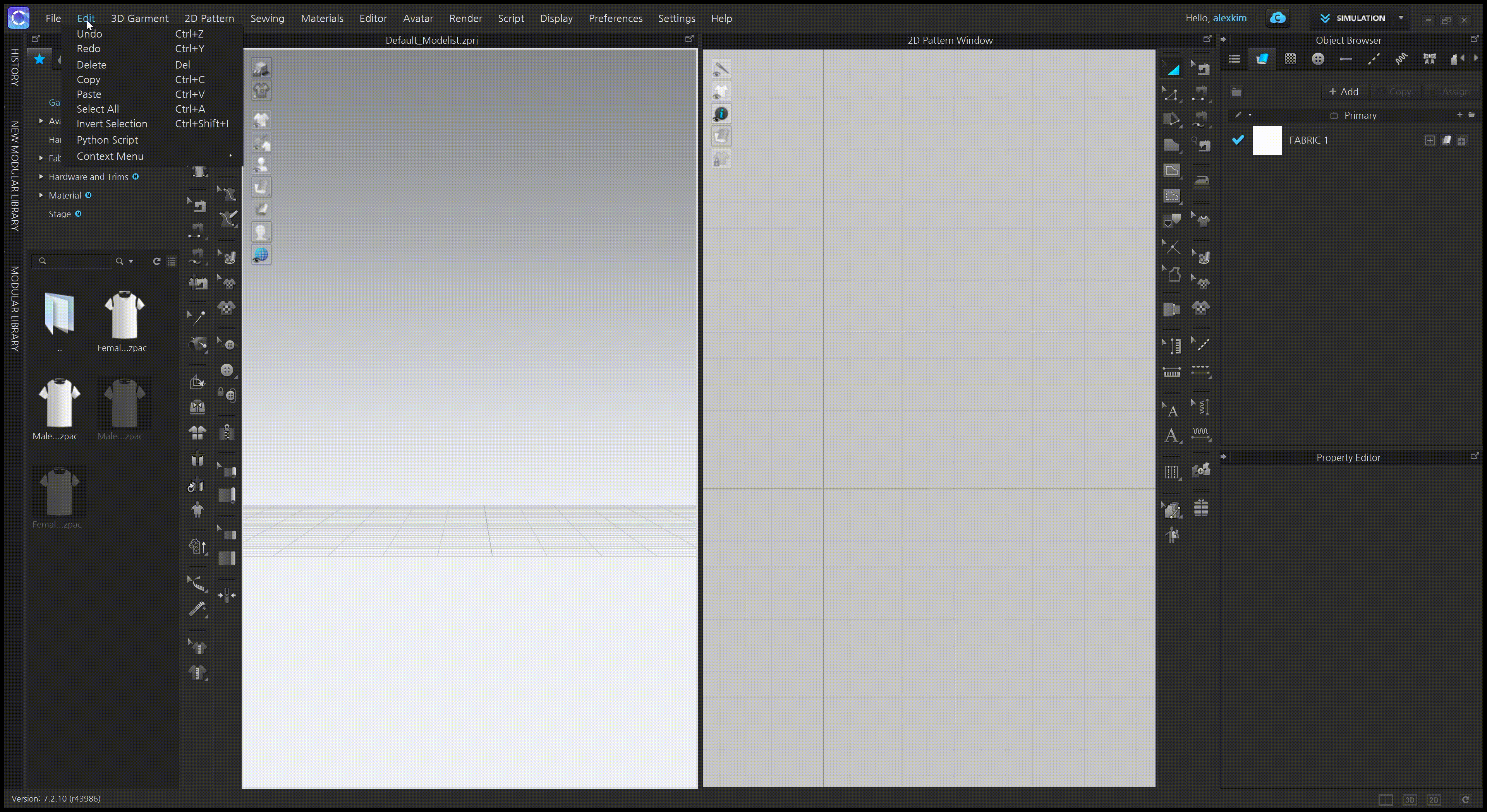
Colorway
import import_api
import utility_api
import fabric_api
print("API Test Scenario - Colorway")
cloAssetFolderPath = "C:/Users/Public/Documents/CLO/Assets"
# Import Cloth File(zpac)
zpacfilePath = cloAssetFolderPath + "/Garment/Female_T-shirt.zpac"
import_api.ImportFile(zpacfilePath)
# set target fabric index
targetFabricIndex = fabric_api.GetFabricCount() - 1
for i in range(3):
# copy last colorway
colorwayCount = utility_api.GetColorwayCount()
copiedColorwayIndex = utility_api.CopyColorway(colorwayCount-1)
# set current colorway
utility_api.SetCurrentColorwayIndex(copiedColorwayIndex)
currentColorwayIndex = utility_api.GetCurrentColorwayIndex()
# set colorway name
alphabet = chr(ord('A') + (i + 1))
colorwayName = "Colorway " + alphabet
print(currentColorwayIndex, colorwayName)
utility_api.SetColorwayName(currentColorwayIndex, colorwayName)
# set diffuse color
# materialFace - 0:Front, 1:Back, 2:Side
r = 0.0
g = 0.0
b = 0.0
if i == 0:
r = 1.0
elif i == 1:
g = 1.0
else:
b = 1.0
fabric_api.SetFabricPBRMaterialBaseColor(currentColorwayIndex, targetFabricIndex, 0, r, g, b, 1.0)
# update colorway
utility_api.UpdateColorways(True)
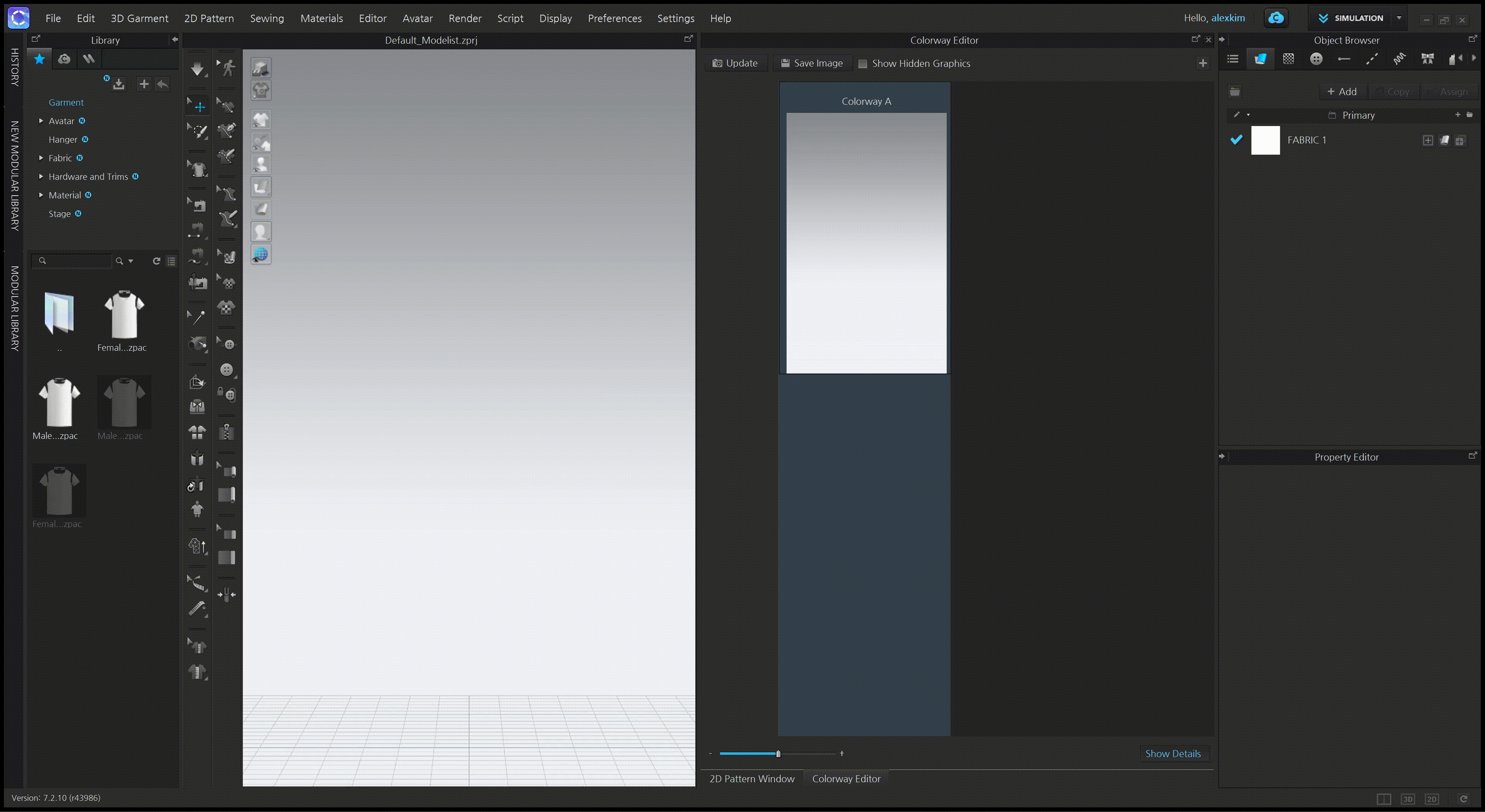
Substance
import import_api
import utility_api
import fabric_api
print("API Test Scenario - Substance")
cloAssetFolderPath = "C:/Users/Public/Documents/CLO/Assets"
# Import Cloth File(zpac)
zpacfilePath = cloAssetFolderPath + "/Garment/Female_T-shirt.zpac"
import_api.ImportFile(zpacfilePath)
# Import Substance
lastFabricIndex = fabric_api.GetFabricCount() - 1
sbsarFilePath = cloAssetFolderPath + "/Materials/Material/Substance/basicjean.sbsar"
fabric_api.ImportSubstanceFileAsFaceType(lastFabricIndex, sbsarFilePath, "Front") # FaceType: Front, Back, Side
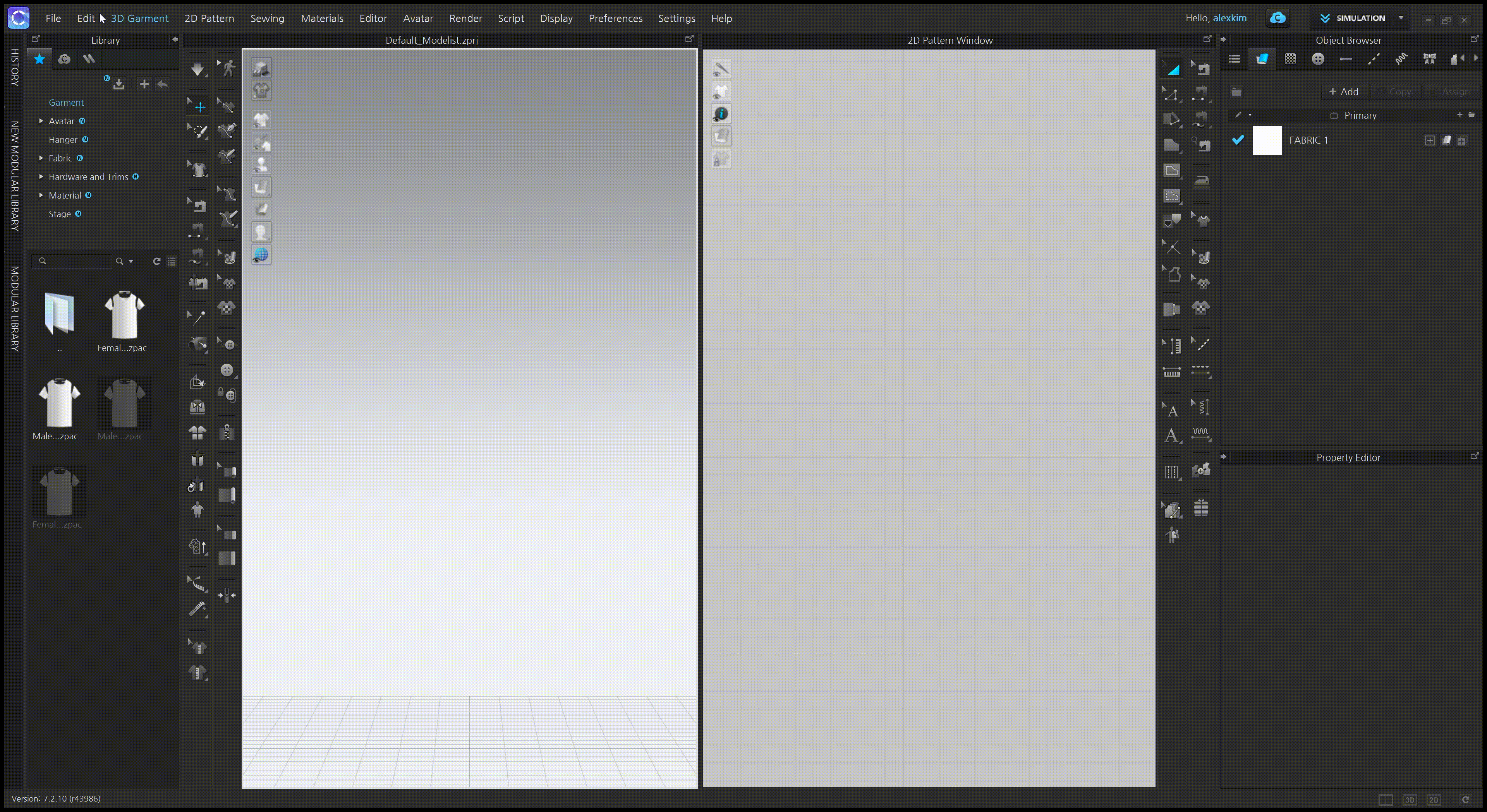
Schematic Render
import import_api
import utility_api
import fabric_api
print("API Test Scenario - Schematic Render")
cloAssetFolderPath = "C:/Users/Public/Documents/CLO/Assets"
# Import Cloth File(zpac)
zpacfilePath = cloAssetFolderPath + "/Garment/Male_T-shirt.zpac"
import_api.ImportFile(zpacfilePath)
#Activate SchematicRender
utility_api.SetSchematicRender(True)
#Deactivate SchematicRender
utility_api.SetSchematicRender(False)
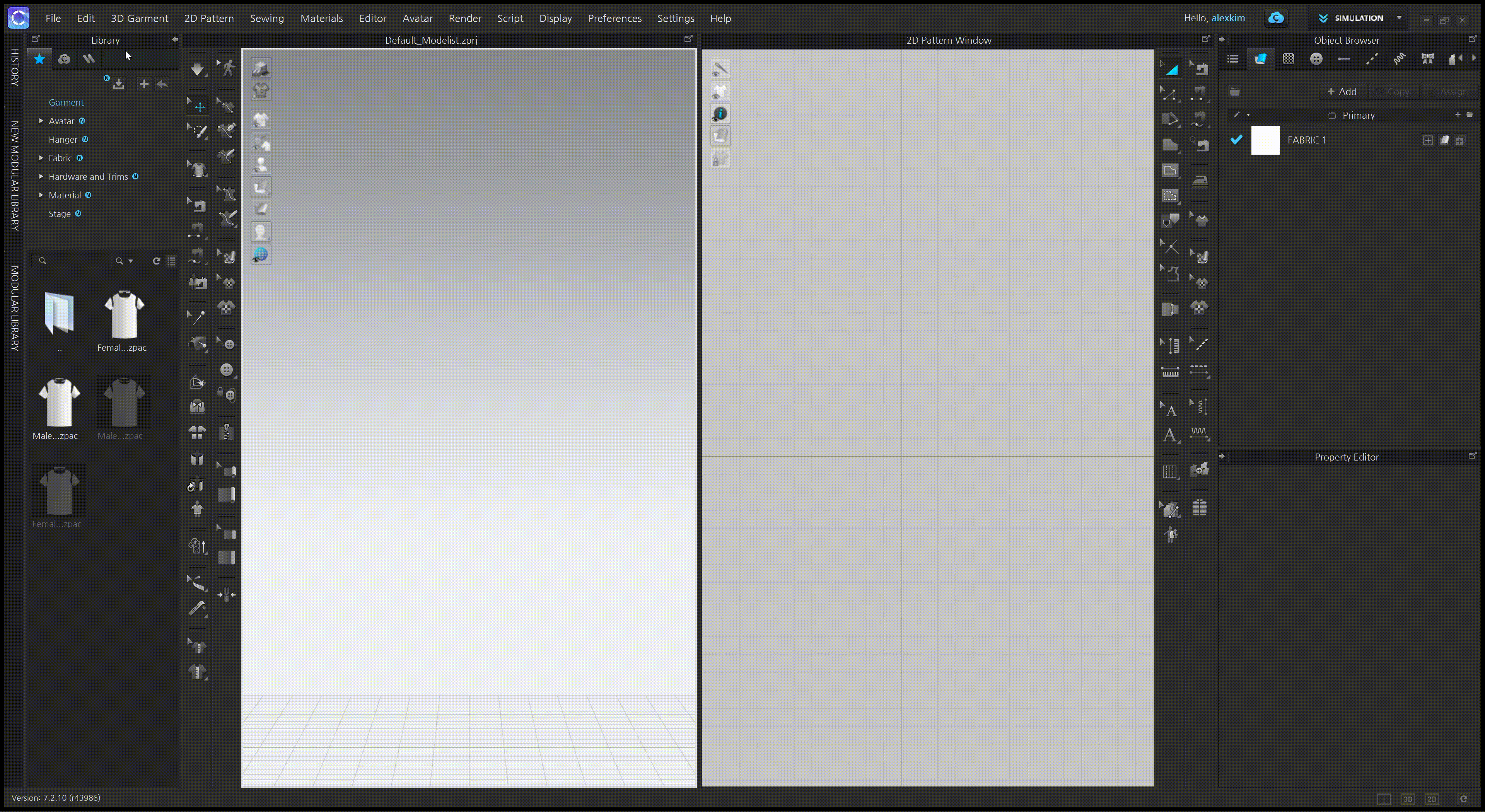